In this blog post, we’ll show you how to Build a Blazor .NET App that Recognizes Images with OpenAI.
You’ll see how we securely upload image files, send them to OpenAI’s API, and return a natural-language response—seamlessly integrated into a modern web interface. This example highlights how CPI Consulting applies advanced AI to solve real-world business challenges through scalable, production-ready .NET solutions.
At CPI Consulting, we specialize in building modern .NET applications integrated with cutting-edge AI. In this post, we’ll walk through how our internal Blazor Server app uses OpenAI’s new GPT-4o model to describe image content—demonstrating what’s possible when AI meets enterprise-grade development.
This solution uploads an image, sends it to OpenAI, and returns a natural-language description of what’s inside—all from a user-friendly web interface.
To see this application in action watch the YouTube video of this post, Building a Blazor .NET App that Recognizes Images with OpenAI.
🧠 How It Works
- A user selects an image in the Blazor UI.
- The C# code uploads the image to OpenAI using the API.
- A GPT-4o model processes the image and returns descriptive text.
- The Blazor app displays the result.
This is powered by OpenAI’s multimodal capability—GPT-4o can interpret both text and images together in one context window.
🔧 Key Components and Code
File Upload to OpenAI
To handle file uploads, we use OpenAI’s official .NET SDK and wrap it in a reusable service. The snippet below shows how a file stream is uploaded using the Vision-specific FileUploadPurpose
.
var fileUploadResult = await fileClient.UploadFileAsync(
imageStream,
"image.jpg",
FileUploadPurpose.Vision
);
We make sure to validate the file before uploading and catch exceptions for resilience in production scenarios.
Sending Image to GPT-4o
After uploading, the image is passed to the GPT-4o model using OpenAIResponseClient
. We combine both text and image inputs into the same request:
var inputItems = new List
{
ResponseItem.CreateUserMessageItem(new[]
{
ResponseContentPart.CreateInputTextPart("What is in this image?"),
ResponseContentPart.CreateInputImagePart(uploadedFile.Id)
})
};
The model responds with structured content:
foreach (var outputItem in response.OutputItems)
{
if (outputItem is MessageResponseItem message)
return message.Content.FirstOrDefault()?.Text ?? "No response.";
}
💡 Blazor Integration
On the frontend, we use InputFile
to handle user uploads. The selected image is streamed directly into memory and passed to the backend service:
private async Task OnInputFileChange(InputFileChangeEventArgs e)
{
using var stream = e.File.OpenReadStream();
ResponseText = await imageService.RecognizeImageAsync(stream);
}
This approach keeps the app lightweight, scalable, and secure. Below you can see the Blazor interface.
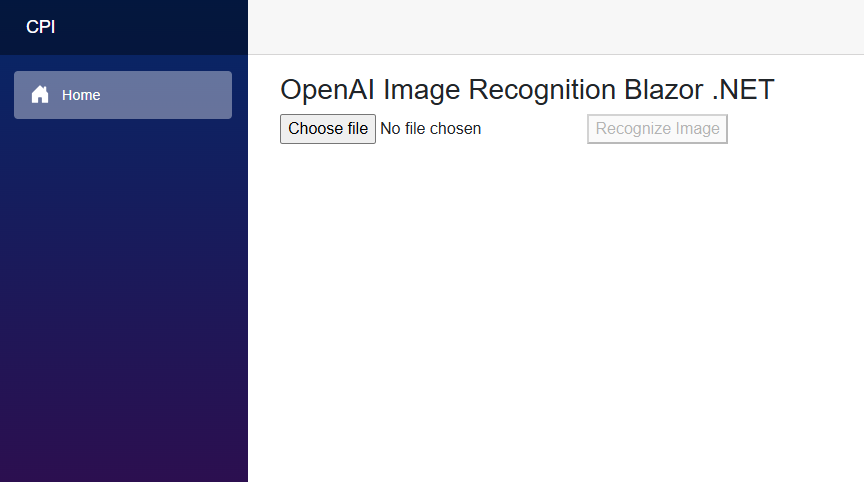
Once uploading an image OpenAI gpt-4o retunrs the object description
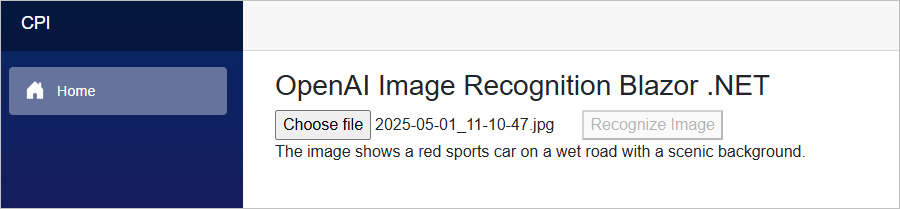
🌍 Use Cases
- Retail & eCommerce: Automated product tagging.
- Security & Compliance: Flagging restricted content in uploads.
- Accessibility: Generating alt-text for screen readers.
- Legal: Describing evidence or document images.
Let’s Build It Together
Need to add vision capabilities to your .NET applications? Contact CPI Consulting and let’s build something powerful.
Discover more from CPI Consulting Pty Ltd Experts in Cloud, AI and Cybersecurity
Subscribe to get the latest posts sent to your email.